Akka tutorials: Messages between actors v.1
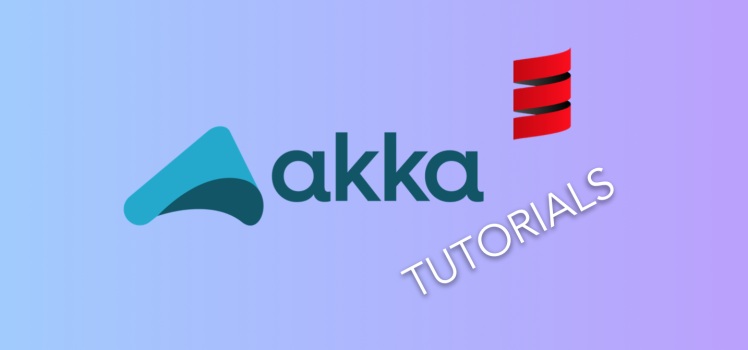
Probably the most popular process which happens behind the scenes in Akka is a messaging. And
it’s not, what comes to mind instantly, no any text messages like in Skype or mobile phones. Akka Actors can deal with different objects and classes which represent messages (Strings also allowed). As usually I’ll use Scala as a programming language.
In this blog post I want to show how to send non-trivial messages between Actors. By saying non-trivial I mean something what differs from String
messages, because for the String
I’ve already published the example. There are 2 possible cases here:
1. object
2. case class
For instance you want to create an Actor which sorts a List of persons. Then, you send a list of persons as a message to this Actor. What happens next? The Actor handles it.
Let’s see on practice how we can use case classes and objects as messages.
Send message to Actor
In this section I’m going to show how to send a message to Actor
from so called “non Actor”. That’s means we are sending a message from outside of Actor System. As a consequence, such messages will have deadLetters
as sender.
Let’s declare a new Actor
with name DeveloperActor
.
import akka.actor.Actor import akka.event.Logging class DeveloperActor extends Actor { import DeveloperActor._ val log = Logging.getLogger(context.system, this) def receive = { case feature: NewFeature => log.info(s"Working on feature '${feature.name}'") case Bug => log.info("Fixing a bug") case _ => log.info("Playing StarCraft2") } }
Depending on message, the DeveloperActor
can do three things:
a) Work on a new feature
b) Fix a bug
c) Play StarCraft2
What about messages? We need to declare them somewhere, otherwise IDE will underline the code, saying that it can’t find the classes. Usually messages related to a particular Actor
, are stored in a companion object of this Actor
.
import akka.actor.Props object DeveloperActor { def props = Props(new DeveloperActor) case class NewFeature(name: String) object Bug }
Also you probably noticed the props
factory method. It provides Props
for the DeveloperActor
creation. This approach is very convenient, because it keeps Actor’s properties in one place and can be used any time.
Everything is ready for the first demonstration:
import akka.actor.ActorSystem import com.actor.DeveloperActor.{Bug, NewFeature} object Demo { def main(args: Array[String]): Unit = { val system = ActorSystem.create("outsource-company") val developer = system.actorOf(DeveloperActor.props, "Bob") developer ! NewFeature("Social integration") developer ! Bug system.terminate() } }
In the code above, I send two different messages to the DeveloperActor
. In its turn it handles them appropriately. Try to run the code locally to see the results.
Send message to Actor from Actor
Akka allow a communication between Actors. Doesn’t matter, we want to send a message to Actor’s children or to siblings, the mechanism of messaging remains constant.
Let’s create one more Actor
:
import akka.actor.{Props, Actor} import akka.event.Logging import com.actor.DeveloperActor.NewFeature class TesterActor extends Actor { val log = Logging.getLogger(context.system, this) def receive = { case feature: NewFeature => log.info(s"Testing '${feature.name}'...") case _ => log.info("Watching YouTube") } } object TesterActor { def props = Props(new TesterActor) }
Now we can send a message from the DeveloperActor
to the TesterActor
:
import akka.actor.ActorSystem import com.actor.DeveloperActor.NewFeature object Demo { def main(args: Array[String]): Unit = { val system = ActorSystem.create("outsource-company") val developer = system.actorOf(DeveloperActor.props, "Bob") val tester = system.actorOf(TesterActor.props, "Sam") developer ! NewFeature("Social integration") tester.tell(NewFeature("Social integration"), developer) system.terminate() } }
With help of the tell
method, I send the NewFeature
message to the TesterActor
. This message will have the developer
as sender. But in general this code isn’t so useful as it can seems. Because Akka doesn’t guarantees that the NewFeature
message will be received by the developer
at first and only after that by the tester
.
In the next post I want to show how to respond to messages, which Actor received from another Actor
.